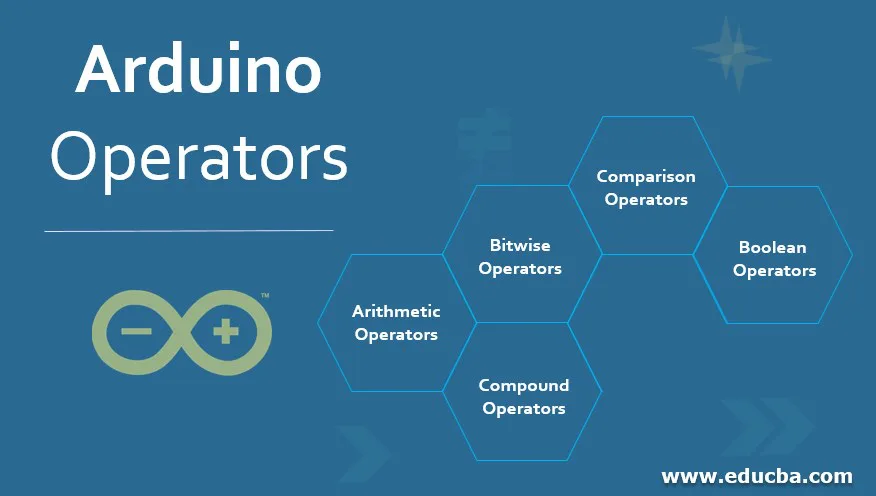
Introduction to Arduino Operators
In Arduino programming, Arduino operator in one of the most important topics. It is used to perform operations like assignment, logical, special, incremental, etc. Knowledge of these operators is important in performing the mathematical and logical computation in Arduino. So basically an operator is responsible for operating on data. For example, if we have to perform the addition of two number then we will be using the addition operator, as it is responsible for performing the addition operation on the data. In Arduino programming, all these operators are grouped in five different categories based upon there operations.
Various Operators used in Arduino Programming
Following are the group of operators that we can use in Arduino programming:
- Arithmetic Operators
- Bitwise Operators
- Comparison Operators
- Boolean Operators
- Compound Operators
1. Arithmetic Operators
Programs are widely used to perform mathematical calculations. In Arduino, we can write simple programs that can perform mathematical computations like addition, subtraction, multiplication, division and many more.
Operator | Symbol | Description | Example |
Assignment Operator | = | It is used to assign the value to the right side of the equal sign to the variable on the left side. | To assign value 10 to B, write B=10. |
Addition Operator | + | It is used to add two operands. | Suppose A=10 and B=20 then A+B will give 30. |
Subtraction Operator | – | It is used to subtract two operands. | Suppose A=30 and B=20 then A-B will give 10. |
Multiplication Operator | * | It is used to multiply operands. | Suppose A=10 and B=2 then A*B will give 20. |
Division Operator | / | It is used to divide two operands. | Suppose A=10 and B=20 then B/A will give 2. |
Modulo Operator | % | It is used to get the remainder of the two operands when they are divided. | Suppose A=10 and B=23 then B%A will give 3. |
2. Comparison Operators
These operators are used to compare the values of the operand. The type of comparisons that are performed are equal, not equal, less than, greater than and many more. Boolean value (True/False) is returned by these comparison operators.
Operator | Symbol | Description | Example |
Equal to Operator | = = | It is used to check if the value of the two operands is equal are not. In the case it is not equal it will return False else it will return True. | Suppose A=20 and B=22 to check if A is equal to B, write A==B. It will return False. |
Not Equal to | != | It is used to check if the value of the two operands is equal are not. In the case it is not equal it will return true else it will return False. | Suppose A=20 and B=22 to check if A is not equal to B, write A!=B. It will return True. |
Less Than | < | It is used to check if the operand on the left side is less than the operand on the right side. In this case, if the condition is valid, it returns true else false. | Suppose A=20 and B=22 to check if A is less than B, write A<B. It will return True. |
Greater Than | > | It is used to check if the operand on the left side is greater than the operand on the right side. In this case, if the condition is valid, it returns true else false. | Suppose A=20 and B=22 to check if A is greater than B, write A>B. It will return False. |
Less than Equal to | <= | It is used to check if the operand on the left side is less than or equal to the operand on the right side. In this case, if the condition is valid, it returns true else false. | Suppose A=20 and B=22 to check if A is less than equal to B, write A<=B. It will return True. |
Greater than equal to | >= | It is used to check if the operand on the left side is greater than or equal to the operand on the right side. In this case, if the condition is valid, it returns true else false. | Suppose A=20 and B=22 to check if A is greater than equal to B, write A>=B. It will return False. |
3. Logical Operators
These operators help take the decision based upon the conditions. In cases where we have to combine the result of two conditions. As a result, these value results in a boolean value.
Operator | Symbol | Description | Example |
Logical And | && | It checks whether both the operands are non-zero or not. In the case if both the operators are non-zero, then the condition becomes valid it will return true else it will return False. | Suppose A is 10 and B is 20 then A&&B is True. |
Logical Or | || | It checks whether either of the two operands is non-zero or not. In the case if either of them is non-zero, then the condition becomes valid, it will return true else it will return False. | Suppose A is 10 and B is 20 then A&&B is True, Suppose A=0 and B=0 then result will be False. |
Logical Not | ! | It changes the logical state of the operands. If the operand is False then not of the operand will result in True. | Suppose A = True then,! A will give False. |
4. Bitwise Operators
These operators perform the operations at the binary level and give the result in the decimal representation. Popular Course in this categoryAll in One Software Development Bundle (600+ Courses, 50+ projects)600+ Online Courses | 3000+ Hours | Verifiable Certificates | Lifetime Access
4.6 (3,144 ratings)Course Price
₹8999 ₹125000
View Course
Related CoursesWindows 10 Training (4 Courses, 4+ Projects)JWS Java Web Services Training (4 Courses, 11 Projects)Java Training (40 Courses, 29 Projects, 4 Quizzes)
Operator | Symbol | Description | Example |
Bitwise AND | & | It increases the value of the operand by 1 | Suppose A = 10 and B=20, then A&B will give 2.A = 01010 (10)B = 10100 (20)A&B = 00010 (2) |
Bitwise OR | | | If bits of either of the operands are set it puts a set bit in the result. | Suppose A = 10 and B=20, then A&B will give 30.A = 01010 (10)B = 10100 (20)A|B = 11110 (30) |
Bitwise XOR | ^ | If bits are set only in one operand it is copied to the result. | Suppose A = 42 and B=27, then A^B will give 49.A = 00101010 (42)B = 00011011 (27)A^B = 00110001 (49) |
Bitwise NOT | ~ | It is a binary operator, it flips the bits of the operand. | Suppose A =10 then ~A will give 21.A = 01010 (10)~A = 10101 (21) |
Shift Left | << | The bits of the operand on the left side of the shift left operator is moved towards the left side based upon the value of the operand on the right side of the shift left operator. | Suppose A = 42 and B=2, then A<<B will give 168.A = 00101010 (42)A<<B = 10101000 (49) |
Shift Right | >> | The bits of the operand on the left side of the shift right operator is moved towards the right side based upon the value of the operand on the right side of the shift right operator. | Suppose A = 42 and B=2, then A>>B will give 10.A = 00101010 (42)A>>B = 00001010 (10) |
5. Compound Operators
Operator | Symbol | Description | Example |
Increment | ++ | It increases the value of the operand by 1. | Suppose A=10 then A++ will give 11 |
Decrement | — | It decreases the value of the operand by 1. | Suppose A=10 then A– will give 9 |
Compound Addition | += | It adds the right operand to the left operand and then stores the result in the left operand. | Suppose A=10 then A+=5 result 15 |
Compound Subtraction | -= | It subtracts the right operand from the left operand and then stores the result in the left operand itself. | Suppose A=10 then A-=5 result 5 |
Compound Multiplication | *= | It multiplies the right operand to the left operand and then stores the result in the left operand. | Suppose A=10 then A*=5 result A=50 |
Compound Division | /= | It multiplies the right operand to the left operand and then stores the result in the left operand. | Suppose A=10 then A*=5 result A=50 |
Compound Modulo | += | It takes modulo of the operands then stores the modulo result in the left operand. | Suppose A=10 then A%=5 result A = 0 |
Compound Addition | += | It adds the right operand to the left operand and then stores the result in the left operand. | Suppose A=10 then A+=5 result A=15 |
Bitwise Inclusive or | != | It takes bitwise or of the right operand with the left operand and then stores the result in the left operand. | Suppose A=10 and B=20 then A !=B will set A=30 |
Bitwise Inclusive and | &= | It takes bitwise and of the right operand with the left operand and then stores the result in the left operand. | Suppose A=10 and B=20 then A &=B will set A=2 |
Conclusion
In this article, we saw the various operators than we can use while doing Arduino programming. You can use these operators to build up your logic, so go ahead and try implementing these operators in the Arduino IDE and run it on your Arduino.
Recommended Articles
This is a guide to Arduino Operators. Here we discuss the basic concept with 5 different operators used in Arduino programming in detail manner. You can also go through our other related articles to learn more –