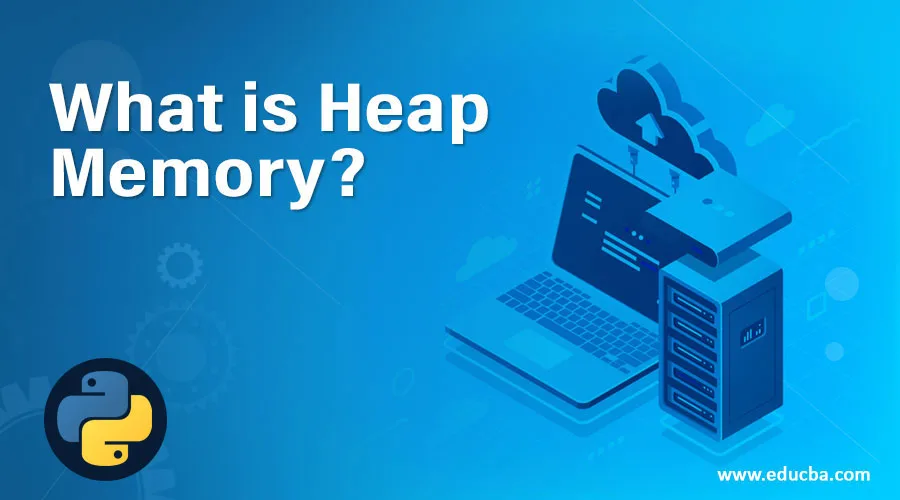
Definition of Heap Memory
Heap memory is a part of memory allocated to JVM, which is shared by all executing threads in the application. It is the part of JVM in which all class instances and are allocated. It is created on the Start-up process of JVM. It does not need to be contiguous, and its size can be static or dynamic. Space allocated to the memory is reclaimed through an automatic memory management process called garbage collection. Heap memory is a shared area that is utilized during the runtime of Java applications. It is created during the instantiation of Java Virtual Machine (JVM).
This memory is shared by instances of all the classes created during the runtime of an application. As per system configuration, the size of heap memory may be fixed or variable. In order to reclaim the space of heap memory, an automatic memory management process called garbage collection is triggered by JVM. JVM provides control to developers to vary the size of heap memory according to requirement.
The functioning of Heap Memory
Java Heap memory is basically divided into two parts:
1. Young Generation
This is a part of java heap memory in which newly created objects are allocated and fully reserved for allocating objects. When space allocated to the young generation gets filled, a process called Minor GC (Garbage collection) is triggered by JVM to clean up space by removing unreferenced objects. The young generation is further divided into the following parts:
- Eden Space: All newly created objects are first allocated to the Eden space of a young generation. It is a larger area of the young generation, and after this Eden space gets filled, Minor GC is triggered, which removes unreferenced objects and moves referenced objects that are survivor objects into another part of the young generation called survivor space.
- Survivor Space: Collection of objects which survived after Minor GC is moved to this area. Survivor space is further subdivided into two parts called survivor to (s0) and survivor from (s1). At the time of Minor GC, objects from one survivor space are moved to other survivor space that is one of the survivor space is always empty. After many cycles of minor GC, survived objects from the young generation are moved to the old generation. Generally, objects are moved to the old generation after crossing the threshold set corresponding to the young generation’s age.
2. Old Generation
Long living objects are moved to the old generation. Objects survived after many cycles of minor GC are considered old enough to be accommodated into this old space. When space in the old generation gets filled, Major GC (Garbage collection) is triggered by JVM to clean up old space resources. Usually, the process of major garbage collection takes more time than minor garbage collection.
If garbage collection is not able to clean space to accommodate new objects, an OutOfMemory error is thrown by JVM. To overcome this error, heap size needs to be increased, or proper memory management is required, which can be done through proper understanding of objects created during the application and which objects are taking more space.
Importance
The importance of heap memory can be summarized using the following points: Popular Course in this categoryJava Training (40 Courses, 29 Projects, 4 Quizzes)40 Online Courses | 29 Hands-on Projects | 285+ Hours | Verifiable Certificate of Completion | Lifetime Access | 4 Quizzes with Solutions
4.8 (9,561 ratings)Course Price
₹7999 ₹41999
View Course
Related CoursesWindows 10 Training (4 Courses, 4+ Projects)JWS Java Web Services Training (4 Courses, 11 Projects)
- With the help of heap memory, we can find the smallest and largest number.
- Garbage collection runs of heap memory to free up space.
- Heaps memory allows global access to variables.
- There is no limit on memory size.
- The priority queue is implemented using heap memory.
Examples of Heap Memory
Following are the different examples of Heap Memory.
Example #1
Now we will see a java example showing how memory is allocated.
Code:
package com.edubca.javademo;
class StudentData {
int rollNumber;
String name;
public StudentData(int rollNumber, String name) {
super();
this.rollNumber = rollNumber;
this.name = name;
}
public int getRollNumber() {
return rollNumber;
}
public void setRollNumber(int rollNumber) {
this.rollNumber = rollNumber;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
public class Main {
public static void main(String[] args) {
int id = 11;
String name = "Yash";
StudentData s = null;
s = new StudentData(id, name);
System.out.println("Student Id is " + s.getRollNumber());
System.out.println("Student Name is " + s.getName());
}
}
Output:
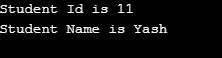
Memory Allocation:
Now we will see how memory is allocated in the above program:
- In the Main class, after entering the main method, since id, the name is local variables a space in stack memory is created in the following way:
- Integer id having primitive value will be stored in stack memory.
- Reference of StudentData object s is stored in stack memory pointing to the original StudentData object, which is stored in heap memory.
- Call to StudentData class constructor will further get added to the top of stack memory. The following will be stored:
- Reference to calling object.
- Integer variable id having value 11.
- Reference of String type variable name which will point to an actual object stored in a string pool in heap memory.
- Two instance variables with the name studentId and studentName declared in StudentData class will be stored in heap memory.
Example #2
Now we will show when JVM throws OutOfMemory error.
Code:
package com.edubca.memoryleak;
public class Main
{
public static void main(String[] args)
{
int[] arr = new int[888888888]; //allocating huge memory
System.out.println("OutOfMemoryError Occurred");
}
}
Output:

The reason for OutOfMemory error is that we are trying to allocate more memory to an integer array than the available space.
Recommended Articles
This is a guide to What is Heap Memory? Here we discuss the definition of Heap Memory, it’s functioning, along with different examples and code implementation. You may also look at the following articles to learn more –
JAVA TRAINING (40 COURSES, 29 PROJECTS, 4 QUIZZES) 40 Online Courses 29 Hands-on Projects 285+ Hours Verifiable Certificate of Completion Lifetime Access 4 Quizzes with SolutionsLearn More0SHARESShareTweetShare