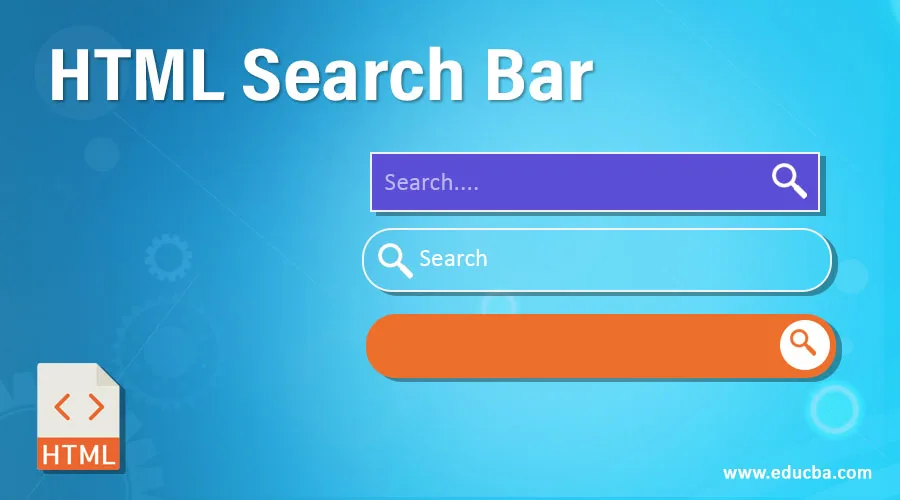
Introduction to HTML Search Bar
Search Bar in HTML is a text field for searching the content if we know the keyword in between the actual sentence. Generally, the search box is always added within the navigation bar. We can also add buttons, drop-down lists, and anchors in the navigation bar as per client requirements, along with the search box. In general, it is put on the top of the page for easy navigation. It will be created by <input class=”form-control” type=”text” placeholder=”Search”>. Based on client requirement, design color varies.
- Real-Time Scenario: Nowadays we are almost all the websites are using navigation bars are having the same.
- Example: https://www.educba.com/ from this website you can see below the search bar.

Why we use CSS in HTML?
Provide common Logic between all the pages; instead of writing the same style logic in each HTML page, we use a CSS file for writing common logic. And include this CSS page in each HTML page with <link> tag.
How does Search Bar work in HTML?
This is used for searching the content by any keyword; if the search keyword found on the page, the result will be displayed. It can be designed with the below syntax.
Syntax:
<div class="navBar">
<a class="active" href="#">Link1</a>
<a href="#">Link2</a>
<a href="#">Link3</a>
<a href="#">Link4</a>
.
.
.
.
<input type="text" placeholder="search here">
</div>
Examples to Implement HTML Search Bar
Below are the examples:
1. Search Bar
Code:
<!DOCTYPE html>
<html>
<head>
<title>Search Bar</title>
<style>
h1 {
text-align: center;
color: blue;
}
* {
outline: none;
}
html, body {
height: 100%;
min-height: 100%;
}
body {
margin: 0;
background-color: brown;
}
.tableBar {
display: table;
width: 100%;
}
.tableDivision {
display: table-cell;
vertical-align: middle;
}
input, button {
margin: 0;
border: 0;
background-color: transparent;
color: green;
font-family: sans-serif;
padding: 0;
}
.top {
position: absolute;
top: 50%;
border-radius: 20px;
transform: scale(0.6);
margin: -83px auto 0 auto;
background-color: white;
left: 0;
right: 0;
width: 550px;
box-shadow: 0 10px 40px aqua, 0 0 0 20px blue;
padding: 35px;
}
form {
height: 96px;
}
input[type="text"] {
font-size: 60px;
line-height: 1;
width: 100%;
height: 96px;
}
input[type="text"]::placeholder {
color: red;
}
#myID {
padding-left: 35px;
width: 1px;
}
button {
width: 84px;
height: 96px;
cursor: pointer;
position: relative;
display: block;
}
#myCircle {
height: 43px;
margin-top: 0;
border-width: 15px;
position: relative;
top: -8px;
border: 15px solid #fff;
background-color: transparent;
border-radius: 50%;
left: 0;
width: 43px;
transition: 0.5s ease all;
background-color: transparent;
border-radius: 50%;
}
button span {
position: absolute;
top: 68px;
border-radius: 10px;
transform: rotateZ(52deg);
transition: 0.5s ease all;
width: 45px;
left: 43px;
display: block;
height: 15px;
background-color: transparent;
display: block;
}
button span:before, button span:after {
content: '';
position: absolute;
background-color: #fff;
border-radius: 10px;
bottom: 0;
right: 0;
transform: rotateZ(0);
transition: 0.5s ease all;
width: 45px;
height: 15px;
transition: 0.5s ease all;
}
#myID:hover #myCircle {
top: -1px;
width: 67px;
height: 15px;
border-width: 0;
background-color: #fff;
border-radius: 20px;
}
#myID:hover span {
top: 50%;
width: 25px;
margin-top: 3px;
left: 56px;
transform: rotateZ(0);
}
#myID:hover button span:before {
transform: rotateZ(52deg);
bottom: 11px;
}
#myID:hover button span:after {
bottom: -11px;
transform: rotateZ(-52deg);
}
#myID:hover button span:before, #myID:hover button span:after {
right: -6px;
width: 40px;
background-color: lime;
}
</style>
</head>
<body>
<h1>Search the Content with Search Bar</h1>
<div class="top">
<form method="get" action="#">
<div class="tableBar">
<div class="tableDivision">
<input type="text" placeholder="Search Here" required>
</div>
<div class="tableDivision" id="myID">
<button type="submit">
<div id="myCircle"></div>
<span></span>
</button>
</div>
</div>
</form>
</div>
</body>
</html>
Output:
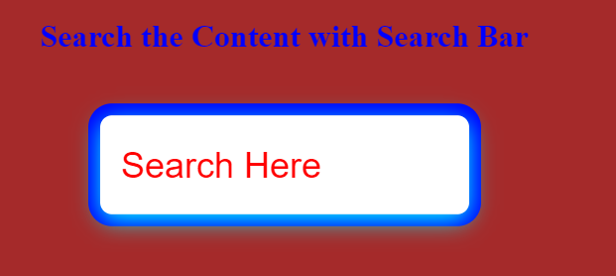
2. Animated Search Bar
Code:
<!DOCTYPE html>
<html>
<head>
<title>Search Bar</title>
<style>
@import url(https://fonts.googleapis.com/css?family=Open+Sans);
body {
background: white;
font-family: fantasy;
}
h1 {
color: red;
text-align: center;
}
.main {
border: solid 2px brown;
font-size: 28px;
color: maroon;
width: 600px;
margin: 150px;
}
.searchTop {
width: 100%;
display: flex;
position: relative;
}
.searchBar {
width: 100%;
border: 3px solid navy;
height: 20px;
border-radius: 5px 0 0 5px;
border-right: none;
padding: 5px;
outline: none;
color: green;
}
.searchBar:focus {
color: purple;
}
.searchButton {
width: 40px;
height: 36px;
font-size: 20px;
text-align: center;
color: white;
border: 1px solid gray;
border-radius: 0 5px 5px 0;
cursor: pointer;
background: gray;
border-radius: 0 5px 5px 0;
cursor: pointer;
}
.topDiv {
width: 30%;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
position: absolute;
left: 50%;
transform: translate(-50%, -50%);
}
</style>
</head>
<body>
<h1>Animated Search Bar with Some Content</h1>
<div class="topDiv">
<div class="searchTop">
<input type="text" class="searchBar"
placeholder="Enter some text to search?">
<button type="submit" class="searchButton">
<i class=""></i>
</button>
</div>
</div>
<div class="main">Now a days we are almost all the websites are
Using navigation bars are having HTML search bar. Example:
https://www.educba.com/.</div>
</body>
</html>
Output:
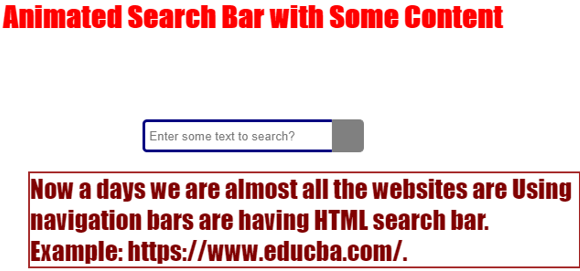
3. Animated Search Bar
Code:
<!DOCTYPE html>
<html>
<head>
<title>Search Bar</title>
<style>
h1
{
color: maroon;
text-align: center;
}
body {
background-image: linear-gradient(to right, green, brown);
}
.topDiv {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-40%, 40%);
height: 40px;
border-radius: 40px;
padding: 10px;
background: #2f3640;
border-radius: 40px;
padding: 10px;
}
.topDiv:hover>.searchBar {
width: 250px;
padding: 0 5px;
}
.topDiv:hover>.button {
background: white;
color: blue;
}
.button {
color: red;
float: right;
height: 45px;
border-radius: 50%;
background: aqua;
width: 45px;
justify-content: center;
align-items: center;
transition: 0.35s;
display: flex;
align-items: center;
transition: 0.35s;
}
.searchBar {
border: none;
background: none;
float: left;
padding: 0;
color: white;
outline: none;
transition: 0.4s;
line-height: 40px;
font-size: 16px;
width: 0px;
line-height: 40px;
padding: 0;
color: white;
}
@media screen and (max-width: 630px) {
.topDiv:hover>.searchBar {
width: 160px;
padding: 0 5px;
}
}
</style>
</head>
<body>
<h1>Animated Search Bar with radius</h1>
<div class="topDiv">
<input class="searchBar" type="text" name="" placeholder="Search">
<button class="button" href="#">
<i class="material-icons"> search </i>
</button>
</div>
</body>
</html>
Output:
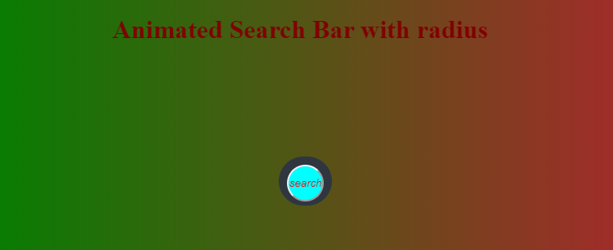
Explanation: When we click move cursor on to the search button, then it can open a text field to enter some content. Popular Course in this categoryHTML Training (12 Courses, 19+ Projects, 4 Quizzes)12 Online Courses | 19 Hands-on Projects | 89+ Hours | Verifiable Certificate of Completion | Lifetime Access | | 4 Quizzes with Solutions
4.5 (6,502 ratings)Course Price
₹6999 ₹41999
View Course
Related CoursesBootstrap Training (2 Courses, 6+ Projects)XML Training (5 Courses, 6+ Projects)CSS Training (9 Courses, 9+ Projects)
Conclusion
It is used for searching the content within the same page or entire page by using different functionality. We can also add some animation and color to the search bar by using CSS properties.
Recommended Articles
This is a guide to HTML Search Bar. Here we discuss how search bar in HTML work with examples to implement with proper codes and outputs. You can also go through our other related articles to learn more –